Note
Go to the end to download the full example code
DiCoDiLe on text images¶
This example illustrates pattern recovery on a noisy text image using DiCoDiLe algorithm.
import matplotlib.pyplot as plt
import numpy as np
from dicodile import dicodile
from dicodile.data.images import fetch_letters_pami
from dicodile.update_d.update_d import tukey_window
from dicodile.utils.csc import reconstruct
from dicodile.utils.dictionary import init_dictionary
from dicodile.utils.viz import display_dictionaries
We will first load PAMI image generated from a text of 5000 characters drawn uniformly from the 4 letters P A M I and 2 whitespaces and assign it to X.
We will also load the images of the four characters used to generate X and assign it to variable D.
X_original, D = fetch_letters_pami()
Downloading data from https://s3-eu-west-1.amazonaws.com/pfigshare-u-files/26750168/text_4_5000_PAMI.npz?X-Amz-Algorithm=AWS4-HMAC-SHA256&X-Amz-Credential=AKIAIYCQYOYV5JSSROOA/20230519/eu-west-1/s3/aws4_request&X-Amz-Date=20230519T144532Z&X-Amz-Expires=10&X-Amz-SignedHeaders=host&X-Amz-Signature=191d18eef2ccf9605e9769e1b71ce3ec5dd9989129c830669fe5a66e3fbfd0f5 (17.8 MB)
file_sizes: 0%| | 0.00/18.6M [00:00<?, ?B/s]
file_sizes: 0%|1 | 73.7k/18.6M [00:00<00:35, 523kB/s]
file_sizes: 1%|3 | 238k/18.6M [00:00<00:20, 897kB/s]
file_sizes: 5%|#3 | 958k/18.6M [00:00<00:06, 2.81MB/s]
file_sizes: 18%|####6 | 3.32M/18.6M [00:00<00:01, 8.26MB/s]
file_sizes: 46%|###########9 | 8.56M/18.6M [00:00<00:00, 18.5MB/s]
file_sizes: 69%|#################8 | 12.8M/18.6M [00:00<00:00, 22.3MB/s]
file_sizes: 100%|##########################| 18.6M/18.6M [00:00<00:00, 28.5MB/s]
file_sizes: 100%|##########################| 18.6M/18.6M [00:00<00:00, 18.7MB/s]
Successfully downloaded file to /github/home/data/dicodile/images/text/text_4_5000_PAMI.npz
We will work on the copy X of the original image and we need to reshape image data X to fit to the expected signal shape of dicodile:
(n_channels, *sig_support)
(1, 2321, 2004)
Reshape D to fit to dictionary format:
(n_atoms, n_channels, *atom_support)
(4, 1, 37, 33)
Let’s display an extract of the original text image X_original and all the images of characters from D.
zoom_x = X_original[190:490, 250:750]
plt.axis('off')
plt.imshow(zoom_x, cmap='gray')
display_dictionaries(D)
<Figure size 640x480 with 4 Axes>
We add some Gaussian white noise with standard deviation std 3 times larger than X.std to X.
We will create a random dictionary of K = 10 patches from the noisy image.
# set number of patches
n_atoms = 10
# set individual atom (patch) size
atom_support = np.array(D.shape[-2:])
D_init = init_dictionary(X, n_atoms=n_atoms, atom_support=atom_support,
random_state=60)
# window the dictionary, this helps make sure that the border values are 0
atom_support = D_init.shape[-2:]
tw = tukey_window(atom_support)[None, None]
D_init *= tw
print(D_init.shape)
(10, 1, 37, 33)
Let’s display an extract of noisy X and random dictionary D_init generated from X.
<Figure size 640x480 with 12 Axes>
Set model parameters.
# regularization parameter
reg = .2
# maximum number of iterations
n_iter = 100
# when True, makes sure that the borders of the atoms are 0
window = True
# when True, requires all activations Z to be positive
z_positive = True
# number of workers to be used for computations
n_workers = 10
# number of jobs per row
w_world = 'auto'
# tolerance for minimal update size
tol = 1e-3
Fit the dictionary with dicodile.
[DEBUG:DICODILE] Lambda_max = 23.816136424875115
Started 10 workers in 4.22s
[INFO:DICODILE] - CD iterations 0 / 100 (0s)
[DEBUG:DICODILE] lambda = 4.763e+00
[INFO:DICOD-10] converged in 9.692s (7.917s) with 105507 iterations (13831 updates).
[DEBUG:DICODILE] Objective (z) : 3.322e+06 (12s)
[PROGRESS:Update D] 2s - 1.00% iterations (1.321e+00)
[PROGRESS:Update D] 4s - 2.00% iterations (2.180e-03)
[PROGRESS:Update D] 4s - 3.00% iterations (1.089e-03)
[PROGRESS:Update D] 5s - 4.00% iterations (1.089e-03)
[PROGRESS:Update D] 5s - 5.00% iterations (1.089e-03)
[PROGRESS:Update D] 6s - 6.00% iterations (1.089e-03)
[PROGRESS:Update D] 6s - 7.00% iterations (1.089e-03)
[PROGRESS:Update D] 6s - 8.00% iterations (1.089e-03)
[PROGRESS:Update D] 7s - 9.00% iterations (1.089e-03)
[PROGRESS:Update D] 7s - 10.00% iterations (1.089e-03)
[PROGRESS:Update D] 8s - 11.00% iterations (1.089e-03)
[PROGRESS:Update D] 8s - 12.00% iterations (1.089e-03)
[PROGRESS:Update D] 8s - 13.00% iterations (1.089e-03)
[PROGRESS:Update D] 9s - 14.00% iterations (1.089e-03)
[PROGRESS:Update D] 9s - 15.00% iterations (1.089e-03)
[PROGRESS:Update D] 9s - 16.00% iterations (1.089e-03)
[PROGRESS:Update D] 10s - 17.00% iterations (1.089e-03)
[PROGRESS:Update D] 10s - 18.00% iterations (1.089e-03)
[PROGRESS:Update D] 11s - 19.00% iterations (1.089e-03)
[PROGRESS:Update D] 11s - 20.00% iterations (2.301e-04)
[INFO:Update D]: 21 iterations
[DEBUG:DICODILE] Objective (d) : 3.289e+06 (21s)
[INFO:DICODILE] - CD iterations 1 / 100 (39s)
[DEBUG:DICODILE] lambda = 4.763e+00
[INFO:DICOD-10] converged in 105.136s (86.757s) with 768381 iterations (162517 updates).
[DEBUG:DICODILE] Objective (z) : 3.226e+06 (110s)
[PROGRESS:Update D] 3s - 1.00% iterations (2.064e-02)
[PROGRESS:Update D] 4s - 2.00% iterations (2.181e-03)
[PROGRESS:Update D] 5s - 3.00% iterations (1.151e-04)
[PROGRESS:Update D] 6s - 4.00% iterations (5.751e-05)
[PROGRESS:Update D] 6s - 5.00% iterations (5.751e-05)
[PROGRESS:Update D] 6s - 6.00% iterations (5.751e-05)
[PROGRESS:Update D] 7s - 7.00% iterations (5.751e-05)
[PROGRESS:Update D] 7s - 8.00% iterations (5.751e-05)
[PROGRESS:Update D] 8s - 9.00% iterations (5.751e-05)
[PROGRESS:Update D] 8s - 10.00% iterations (5.751e-05)
[PROGRESS:Update D] 8s - 11.00% iterations (5.751e-05)
[PROGRESS:Update D] 9s - 12.00% iterations (5.751e-05)
[PROGRESS:Update D] 9s - 13.00% iterations (5.751e-05)
[PROGRESS:Update D] 10s - 14.00% iterations (2.875e-05)
[PROGRESS:Update D] 10s - 15.00% iterations (2.875e-05)
[PROGRESS:Update D] 11s - 16.00% iterations (1.438e-05)
[PROGRESS:Update D] 11s - 17.00% iterations (1.438e-05)
[PROGRESS:Update D] 12s - 18.00% iterations (1.438e-05)
[PROGRESS:Update D] 12s - 19.00% iterations (1.438e-05)
[PROGRESS:Update D] 12s - 20.00% iterations (1.438e-05)
[PROGRESS:Update D] 13s - 21.00% iterations (1.438e-05)
[PROGRESS:Update D] 13s - 22.00% iterations (1.438e-05)
[PROGRESS:Update D] 13s - 23.00% iterations (1.438e-05)
[PROGRESS:Update D] 14s - 24.00% iterations (1.438e-05)
[PROGRESS:Update D] 14s - 25.00% iterations (1.438e-05)
[PROGRESS:Update D] 15s - 26.00% iterations (1.438e-05)
[PROGRESS:Update D] 15s - 27.00% iterations (1.438e-05)
[PROGRESS:Update D] 15s - 28.00% iterations (1.438e-05)
[PROGRESS:Update D] 16s - 29.00% iterations (1.438e-05)
[PROGRESS:Update D] 16s - 30.00% iterations (1.438e-05)
[PROGRESS:Update D] 17s - 31.00% iterations (1.438e-05)
[PROGRESS:Update D] 17s - 32.00% iterations (1.438e-05)
[PROGRESS:Update D] 17s - 33.00% iterations (1.438e-05)
[PROGRESS:Update D] 18s - 34.00% iterations (1.438e-05)
[PROGRESS:Update D] 18s - 35.00% iterations (1.438e-05)
[PROGRESS:Update D] 19s - 36.00% iterations (1.438e-05)
[PROGRESS:Update D] 19s - 37.00% iterations (1.438e-05)
[INFO:Update D]: 38 iterations
[DEBUG:DICODILE] Objective (d) : 3.218e+06 (29s)
[INFO:DICODILE] - CD iterations 2 / 100 (185s)
[DEBUG:DICODILE] lambda = 4.763e+00
[INFO:DICOD-10] converged in 98.236s (80.487s) with 729887 iterations (154125 updates).
[DEBUG:DICODILE] Objective (z) : 3.209e+06 (105s)
[PROGRESS:Update D] 3s - 1.00% iterations (1.032e-02)
[PROGRESS:Update D] 4s - 2.00% iterations (5.159e-03)
[PROGRESS:Update D] 5s - 3.00% iterations (1.363e-04)
[PROGRESS:Update D] 6s - 4.00% iterations (6.812e-05)
[PROGRESS:Update D] 6s - 5.00% iterations (6.812e-05)
[PROGRESS:Update D] 6s - 6.00% iterations (6.812e-05)
[PROGRESS:Update D] 7s - 7.00% iterations (6.812e-05)
[PROGRESS:Update D] 7s - 8.00% iterations (3.406e-05)
[PROGRESS:Update D] 8s - 9.00% iterations (3.406e-05)
[PROGRESS:Update D] 8s - 10.00% iterations (3.406e-05)
[PROGRESS:Update D] 9s - 11.00% iterations (3.406e-05)
[PROGRESS:Update D] 9s - 12.00% iterations (1.703e-05)
[PROGRESS:Update D] 10s - 13.00% iterations (1.703e-05)
[INFO:Update D]: 14 iterations
[DEBUG:DICODILE] Objective (d) : 3.206e+06 (20s)
[INFO:DICODILE] - CD iterations 3 / 100 (316s)
[DEBUG:DICODILE] lambda = 4.763e+00
[INFO:DICOD-10] converged in 84.464s (69.503s) with 492200 iterations (137070 updates).
[DEBUG:DICODILE] Objective (z) : 3.200e+06 (91s)
[PROGRESS:Update D] 3s - 1.00% iterations (2.064e-02)
[PROGRESS:Update D] 3s - 2.00% iterations (1.032e-02)
[PROGRESS:Update D] 5s - 3.00% iterations (6.810e-05)
[PROGRESS:Update D] 5s - 4.00% iterations (6.810e-05)
[PROGRESS:Update D] 6s - 5.00% iterations (3.404e-05)
[PROGRESS:Update D] 6s - 6.00% iterations (3.404e-05)
[PROGRESS:Update D] 7s - 7.00% iterations (3.404e-05)
[PROGRESS:Update D] 7s - 8.00% iterations (3.404e-05)
[PROGRESS:Update D] 8s - 9.00% iterations (1.702e-05)
[PROGRESS:Update D] 8s - 10.00% iterations (1.702e-05)
[PROGRESS:Update D] 9s - 11.00% iterations (1.702e-05)
[PROGRESS:Update D] 9s - 12.00% iterations (1.702e-05)
[PROGRESS:Update D] 9s - 13.00% iterations (1.702e-05)
[PROGRESS:Update D] 10s - 14.00% iterations (1.702e-05)
[PROGRESS:Update D] 10s - 15.00% iterations (1.702e-05)
[PROGRESS:Update D] 11s - 16.00% iterations (1.702e-05)
[PROGRESS:Update D] 11s - 17.00% iterations (1.702e-05)
[PROGRESS:Update D] 11s - 18.00% iterations (1.702e-05)
[PROGRESS:Update D] 12s - 19.00% iterations (1.702e-05)
[PROGRESS:Update D] 12s - 20.00% iterations (1.702e-05)
[PROGRESS:Update D] 13s - 21.00% iterations (1.702e-05)
[PROGRESS:Update D] 13s - 22.00% iterations (1.702e-05)
[PROGRESS:Update D] 13s - 23.00% iterations (1.702e-05)
[PROGRESS:Update D] 14s - 24.00% iterations (1.702e-05)
[PROGRESS:Update D] 14s - 25.00% iterations (1.702e-05)
[PROGRESS:Update D] 15s - 26.00% iterations (1.702e-05)
[PROGRESS:Update D] 15s - 27.00% iterations (1.702e-05)
[PROGRESS:Update D] 15s - 28.00% iterations (1.702e-05)
[PROGRESS:Update D] 16s - 29.00% iterations (1.702e-05)
[PROGRESS:Update D] 16s - 30.00% iterations (1.702e-05)
[PROGRESS:Update D] 16s - 31.00% iterations (1.702e-05)
[PROGRESS:Update D] 17s - 32.00% iterations (1.702e-05)
[PROGRESS:Update D] 17s - 33.00% iterations (1.702e-05)
[PROGRESS:Update D] 18s - 34.00% iterations (1.702e-05)
[PROGRESS:Update D] 18s - 35.00% iterations (1.702e-05)
[PROGRESS:Update D] 18s - 36.00% iterations (1.702e-05)
[PROGRESS:Update D] 19s - 37.00% iterations (1.702e-05)
[PROGRESS:Update D] 19s - 38.00% iterations (1.702e-05)
[PROGRESS:Update D] 20s - 39.00% iterations (1.702e-05)
[PROGRESS:Update D] 20s - 40.00% iterations (1.702e-05)
[PROGRESS:Update D] 20s - 41.00% iterations (1.702e-05)
[PROGRESS:Update D] 21s - 42.00% iterations (1.702e-05)
[PROGRESS:Update D] 21s - 43.00% iterations (1.702e-05)
[PROGRESS:Update D] 22s - 44.00% iterations (1.702e-05)
[PROGRESS:Update D] 22s - 45.00% iterations (1.702e-05)
[PROGRESS:Update D] 22s - 46.00% iterations (1.702e-05)
[PROGRESS:Update D] 23s - 47.00% iterations (1.702e-05)
[PROGRESS:Update D] 23s - 48.00% iterations (1.702e-05)
[PROGRESS:Update D] 23s - 49.00% iterations (1.702e-05)
[PROGRESS:Update D] 24s - 50.00% iterations (1.702e-05)
[PROGRESS:Update D] 24s - 51.00% iterations (1.702e-05)
[PROGRESS:Update D] 25s - 52.00% iterations (1.702e-05)
[PROGRESS:Update D] 25s - 53.00% iterations (1.702e-05)
[PROGRESS:Update D] 25s - 54.00% iterations (1.702e-05)
[PROGRESS:Update D] 26s - 55.00% iterations (1.702e-05)
[PROGRESS:Update D] 26s - 56.00% iterations (1.702e-05)
[PROGRESS:Update D] 27s - 57.00% iterations (1.702e-05)
[PROGRESS:Update D] 27s - 58.00% iterations (1.702e-05)
[PROGRESS:Update D] 27s - 59.00% iterations (1.702e-05)
[PROGRESS:Update D] 28s - 60.00% iterations (1.702e-05)
[PROGRESS:Update D] 28s - 61.00% iterations (1.702e-05)
[PROGRESS:Update D] 29s - 62.00% iterations (1.702e-05)
[PROGRESS:Update D] 29s - 63.00% iterations (1.702e-05)
[PROGRESS:Update D] 29s - 64.00% iterations (1.702e-05)
[INFO:Update D]: 65 iterations
[DEBUG:DICODILE] Objective (d) : 3.195e+06 (40s)
[INFO:DICODILE] - CD iterations 4 / 100 (453s)
[DEBUG:DICODILE] lambda = 4.763e+00
[INFO:DICOD-10] converged in 73.848s (62.045s) with 591172 iterations (117856 updates).
[DEBUG:DICODILE] Objective (z) : 3.187e+06 (80s)
[PROGRESS:Update D] 3s - 1.00% iterations (4.127e-02)
[PROGRESS:Update D] 4s - 2.00% iterations (2.181e-03)
[PROGRESS:Update D] 5s - 3.00% iterations (5.756e-05)
[PROGRESS:Update D] 6s - 4.00% iterations (5.756e-05)
[PROGRESS:Update D] 6s - 5.00% iterations (2.877e-05)
[PROGRESS:Update D] 6s - 6.00% iterations (2.877e-05)
[PROGRESS:Update D] 7s - 7.00% iterations (2.877e-05)
[PROGRESS:Update D] 7s - 8.00% iterations (2.877e-05)
[PROGRESS:Update D] 8s - 9.00% iterations (1.438e-05)
[PROGRESS:Update D] 8s - 10.00% iterations (1.438e-05)
[INFO:Update D]: 11 iterations
[DEBUG:DICODILE] Objective (d) : 3.178e+06 (19s)
[INFO:DICODILE] - CD iterations 5 / 100 (558s)
[DEBUG:DICODILE] lambda = 4.763e+00
[INFO:DICOD-10] converged in 69.356s (57.637s) with 419915 iterations (110032 updates).
[DEBUG:DICODILE] Objective (z) : 3.167e+06 (76s)
[PROGRESS:Update D] 4s - 1.00% iterations (1.611e-04)
[PROGRESS:Update D] 5s - 2.00% iterations (8.041e-05)
[PROGRESS:Update D] 5s - 3.00% iterations (4.011e-05)
[PROGRESS:Update D] 6s - 4.00% iterations (4.011e-05)
[PROGRESS:Update D] 6s - 5.00% iterations (4.011e-05)
[PROGRESS:Update D] 7s - 6.00% iterations (4.011e-05)
[PROGRESS:Update D] 7s - 7.00% iterations (2.005e-05)
[PROGRESS:Update D] 8s - 8.00% iterations (2.005e-05)
[INFO:Update D]: 9 iterations
[DEBUG:DICODILE] Objective (d) : 3.157e+06 (18s)
[INFO:DICODILE] - CD iterations 6 / 100 (658s)
[DEBUG:DICODILE] lambda = 4.763e+00
[INFO:DICOD-10] converged in 60.628s (52.248s) with 396445 iterations (94710 updates).
[DEBUG:DICODILE] Objective (z) : 3.151e+06 (67s)
[PROGRESS:Update D] 4s - 1.00% iterations (8.051e-05)
[PROGRESS:Update D] 5s - 2.00% iterations (3.997e-05)
[PROGRESS:Update D] 6s - 3.00% iterations (1.993e-05)
[PROGRESS:Update D] 6s - 4.00% iterations (1.993e-05)
[PROGRESS:Update D] 6s - 5.00% iterations (1.993e-05)
[PROGRESS:Update D] 7s - 6.00% iterations (1.993e-05)
[PROGRESS:Update D] 7s - 7.00% iterations (9.965e-06)
[PROGRESS:Update D] 8s - 8.00% iterations (9.965e-06)
[PROGRESS:Update D] 8s - 9.00% iterations (9.965e-06)
[PROGRESS:Update D] 8s - 10.00% iterations (9.965e-06)
[PROGRESS:Update D] 9s - 11.00% iterations (9.965e-06)
[PROGRESS:Update D] 9s - 12.00% iterations (9.965e-06)
[INFO:Update D]: 13 iterations
[DEBUG:DICODILE] Objective (d) : 3.146e+06 (19s)
[INFO:DICODILE] - CD iterations 7 / 100 (750s)
[DEBUG:DICODILE] lambda = 4.763e+00
[INFO:DICOD-10] converged in 53.584s (45.535s) with 492653 iterations (81762 updates).
[DEBUG:DICODILE] Objective (z) : 3.144e+06 (60s)
[PROGRESS:Update D] 4s - 1.00% iterations (1.612e-04)
[PROGRESS:Update D] 5s - 2.00% iterations (3.377e-05)
[PROGRESS:Update D] 5s - 3.00% iterations (1.686e-05)
[PROGRESS:Update D] 6s - 4.00% iterations (1.686e-05)
[PROGRESS:Update D] 6s - 5.00% iterations (1.686e-05)
[PROGRESS:Update D] 7s - 6.00% iterations (1.686e-05)
[PROGRESS:Update D] 7s - 7.00% iterations (8.432e-06)
[PROGRESS:Update D] 8s - 8.00% iterations (8.432e-06)
[PROGRESS:Update D] 8s - 9.00% iterations (8.432e-06)
[PROGRESS:Update D] 8s - 10.00% iterations (8.432e-06)
[PROGRESS:Update D] 9s - 11.00% iterations (8.432e-06)
[PROGRESS:Update D] 9s - 12.00% iterations (8.432e-06)
[PROGRESS:Update D] 10s - 13.00% iterations (8.432e-06)
[PROGRESS:Update D] 11s - 14.00% iterations (8.909e-07)
[PROGRESS:Update D] 12s - 15.00% iterations (5.884e-09)
[INFO:Update D]: 16 iterations
[DEBUG:DICODILE] Objective (d) : 3.142e+06 (20s)
[INFO:DICODILE] - CD iterations 8 / 100 (835s)
[DEBUG:DICODILE] lambda = 4.763e+00
[INFO:DICOD-10] converged in 39.560s (33.106s) with 266047 iterations (60648 updates).
[DEBUG:DICODILE] Objective (z) : 3.141e+06 (46s)
[PROGRESS:Update D] 1s - 3.00% iterations (1.554e-08)
[PROGRESS:Update D] 2s - 4.00% iterations (1.554e-08)
[PROGRESS:Update D] 2s - 5.00% iterations (1.554e-08)
[PROGRESS:Update D] 2s - 6.00% iterations (1.554e-08)
[PROGRESS:Update D] 3s - 7.00% iterations (1.554e-08)
[PROGRESS:Update D] 3s - 8.00% iterations (1.554e-08)
[PROGRESS:Update D] 4s - 9.00% iterations (1.554e-08)
[PROGRESS:Update D] 4s - 10.00% iterations (1.554e-08)
[PROGRESS:Update D] 4s - 11.00% iterations (1.554e-08)
[PROGRESS:Update D] 5s - 12.00% iterations (1.554e-08)
[PROGRESS:Update D] 5s - 13.00% iterations (1.554e-08)
[PROGRESS:Update D] 6s - 14.00% iterations (1.554e-08)
[PROGRESS:Update D] 6s - 15.00% iterations (1.554e-08)
[PROGRESS:Update D] 6s - 16.00% iterations (1.554e-08)
[PROGRESS:Update D] 7s - 17.00% iterations (1.554e-08)
[PROGRESS:Update D] 7s - 18.00% iterations (1.554e-08)
[PROGRESS:Update D] 7s - 19.00% iterations (1.554e-08)
[PROGRESS:Update D] 8s - 20.00% iterations (1.554e-08)
[PROGRESS:Update D] 8s - 21.00% iterations (1.554e-08)
[PROGRESS:Update D] 9s - 22.00% iterations (1.554e-08)
[PROGRESS:Update D] 9s - 23.00% iterations (1.554e-08)
[PROGRESS:Update D] 9s - 24.00% iterations (1.554e-08)
[PROGRESS:Update D] 10s - 25.00% iterations (1.554e-08)
[PROGRESS:Update D] 10s - 26.00% iterations (1.554e-08)
[PROGRESS:Update D] 11s - 27.00% iterations (1.554e-08)
[PROGRESS:Update D] 11s - 28.00% iterations (1.554e-08)
[PROGRESS:Update D] 11s - 29.00% iterations (1.554e-08)
[PROGRESS:Update D] 12s - 30.00% iterations (1.554e-08)
[PROGRESS:Update D] 12s - 31.00% iterations (1.554e-08)
[PROGRESS:Update D] 13s - 32.00% iterations (1.554e-08)
[PROGRESS:Update D] 13s - 33.00% iterations (1.554e-08)
[PROGRESS:Update D] 13s - 34.00% iterations (1.554e-08)
[PROGRESS:Update D] 14s - 35.00% iterations (1.554e-08)
[PROGRESS:Update D] 14s - 36.00% iterations (1.554e-08)
[PROGRESS:Update D] 15s - 37.00% iterations (1.554e-08)
[PROGRESS:Update D] 15s - 38.00% iterations (1.554e-08)
[PROGRESS:Update D] 15s - 39.00% iterations (1.554e-08)
[PROGRESS:Update D] 16s - 40.00% iterations (1.554e-08)
[PROGRESS:Update D] 16s - 41.00% iterations (1.554e-08)
[PROGRESS:Update D] 17s - 42.00% iterations (1.554e-08)
[PROGRESS:Update D] 17s - 43.00% iterations (1.554e-08)
[PROGRESS:Update D] 17s - 44.00% iterations (1.554e-08)
[PROGRESS:Update D] 18s - 45.00% iterations (1.554e-08)
[PROGRESS:Update D] 18s - 46.00% iterations (1.554e-08)
[PROGRESS:Update D] 19s - 47.00% iterations (1.554e-08)
[PROGRESS:Update D] 19s - 48.00% iterations (1.554e-08)
[PROGRESS:Update D] 19s - 49.00% iterations (1.554e-08)
[PROGRESS:Update D] 20s - 50.00% iterations (1.554e-08)
[PROGRESS:Update D] 20s - 51.00% iterations (1.554e-08)
[PROGRESS:Update D] 21s - 52.00% iterations (1.554e-08)
[PROGRESS:Update D] 21s - 53.00% iterations (1.554e-08)
[PROGRESS:Update D] 21s - 54.00% iterations (1.554e-08)
[PROGRESS:Update D] 22s - 55.00% iterations (1.554e-08)
[PROGRESS:Update D] 22s - 56.00% iterations (1.554e-08)
[PROGRESS:Update D] 23s - 57.00% iterations (1.554e-08)
[PROGRESS:Update D] 23s - 58.00% iterations (1.554e-08)
[PROGRESS:Update D] 23s - 59.00% iterations (1.554e-08)
[PROGRESS:Update D] 24s - 60.00% iterations (1.554e-08)
[PROGRESS:Update D] 24s - 61.00% iterations (1.554e-08)
[PROGRESS:Update D] 24s - 62.00% iterations (1.554e-08)
[PROGRESS:Update D] 25s - 63.00% iterations (1.554e-08)
[PROGRESS:Update D] 25s - 64.00% iterations (1.554e-08)
[PROGRESS:Update D] 26s - 65.00% iterations (1.554e-08)
[PROGRESS:Update D] 26s - 66.00% iterations (1.554e-08)
[PROGRESS:Update D] 26s - 67.00% iterations (1.554e-08)
[PROGRESS:Update D] 27s - 68.00% iterations (1.554e-08)
[PROGRESS:Update D] 27s - 69.00% iterations (1.554e-08)
[PROGRESS:Update D] 28s - 70.00% iterations (1.554e-08)
[PROGRESS:Update D] 28s - 71.00% iterations (1.554e-08)
[PROGRESS:Update D] 28s - 72.00% iterations (1.554e-08)
[PROGRESS:Update D] 29s - 73.00% iterations (1.554e-08)
[PROGRESS:Update D] 29s - 74.00% iterations (1.554e-08)
[PROGRESS:Update D] 30s - 75.00% iterations (1.554e-08)
[PROGRESS:Update D] 30s - 76.00% iterations (1.554e-08)
[PROGRESS:Update D] 30s - 77.00% iterations (1.554e-08)
[PROGRESS:Update D] 31s - 78.00% iterations (1.554e-08)
[PROGRESS:Update D] 31s - 79.00% iterations (1.554e-08)
[PROGRESS:Update D] 32s - 80.00% iterations (1.554e-08)
[PROGRESS:Update D] 32s - 81.00% iterations (1.554e-08)
[PROGRESS:Update D] 32s - 82.00% iterations (1.554e-08)
[PROGRESS:Update D] 33s - 83.00% iterations (1.554e-08)
[PROGRESS:Update D] 33s - 84.00% iterations (1.554e-08)
[PROGRESS:Update D] 34s - 85.00% iterations (1.554e-08)
[PROGRESS:Update D] 34s - 86.00% iterations (1.554e-08)
[PROGRESS:Update D] 34s - 87.00% iterations (1.554e-08)
[PROGRESS:Update D] 35s - 88.00% iterations (1.554e-08)
[PROGRESS:Update D] 35s - 89.00% iterations (1.554e-08)
[PROGRESS:Update D] 36s - 90.00% iterations (1.554e-08)
[PROGRESS:Update D] 36s - 91.00% iterations (1.554e-08)
[PROGRESS:Update D] 36s - 92.00% iterations (1.554e-08)
[PROGRESS:Update D] 37s - 93.00% iterations (1.554e-08)
[PROGRESS:Update D] 37s - 94.00% iterations (1.554e-08)
[PROGRESS:Update D] 37s - 95.00% iterations (1.554e-08)
[PROGRESS:Update D] 38s - 96.00% iterations (1.554e-08)
[PROGRESS:Update D] 38s - 97.00% iterations (1.554e-08)
[PROGRESS:Update D] 39s - 98.00% iterations (1.554e-08)
[PROGRESS:Update D] 39s - 99.00% iterations (1.554e-08)
[INFO:Update D] update did not converge
[INFO:Update D]: 100 iterations
[DEBUG:DICODILE] Objective (d) : 3.141e+06 (45s)
[INFO:DICODILE] - CD iterations 9 / 100 (932s)
[DEBUG:DICODILE] lambda = 4.763e+00
[INFO:DICOD-10] converged in 6.856s (5.725s) with 62873 iterations (9580 updates).
[DEBUG:DICODILE] Objective (z) : 3.141e+06 (13s)
[PROGRESS:Update D] 1s - 3.00% iterations (1.554e-06)
[PROGRESS:Update D] 2s - 4.00% iterations (1.554e-06)
[PROGRESS:Update D] 2s - 5.00% iterations (1.554e-06)
[PROGRESS:Update D] 2s - 6.00% iterations (1.554e-06)
[PROGRESS:Update D] 3s - 7.00% iterations (1.554e-06)
[PROGRESS:Update D] 3s - 8.00% iterations (1.554e-06)
[PROGRESS:Update D] 4s - 9.00% iterations (1.554e-06)
[PROGRESS:Update D] 4s - 10.00% iterations (1.554e-06)
[PROGRESS:Update D] 4s - 11.00% iterations (1.554e-06)
[PROGRESS:Update D] 5s - 12.00% iterations (1.554e-06)
[PROGRESS:Update D] 5s - 13.00% iterations (1.554e-06)
[PROGRESS:Update D] 5s - 14.00% iterations (1.554e-06)
[PROGRESS:Update D] 6s - 15.00% iterations (1.554e-06)
[PROGRESS:Update D] 6s - 16.00% iterations (1.554e-06)
[PROGRESS:Update D] 7s - 17.00% iterations (1.554e-06)
[PROGRESS:Update D] 7s - 18.00% iterations (1.554e-06)
[PROGRESS:Update D] 7s - 19.00% iterations (1.554e-06)
[PROGRESS:Update D] 8s - 20.00% iterations (1.554e-06)
[PROGRESS:Update D] 8s - 21.00% iterations (1.554e-06)
[PROGRESS:Update D] 9s - 22.00% iterations (1.554e-06)
[PROGRESS:Update D] 9s - 23.00% iterations (1.554e-06)
[PROGRESS:Update D] 9s - 24.00% iterations (1.554e-06)
[PROGRESS:Update D] 10s - 25.00% iterations (1.554e-06)
[PROGRESS:Update D] 10s - 26.00% iterations (1.554e-06)
[PROGRESS:Update D] 11s - 27.00% iterations (1.554e-06)
[PROGRESS:Update D] 11s - 28.00% iterations (1.554e-06)
[PROGRESS:Update D] 11s - 29.00% iterations (1.554e-06)
[PROGRESS:Update D] 12s - 30.00% iterations (1.554e-06)
[PROGRESS:Update D] 12s - 31.00% iterations (1.554e-06)
[PROGRESS:Update D] 13s - 32.00% iterations (1.554e-06)
[PROGRESS:Update D] 13s - 33.00% iterations (1.554e-06)
[PROGRESS:Update D] 13s - 34.00% iterations (1.554e-06)
[PROGRESS:Update D] 14s - 35.00% iterations (1.554e-06)
[PROGRESS:Update D] 14s - 36.00% iterations (1.554e-06)
[PROGRESS:Update D] 15s - 37.00% iterations (1.554e-06)
[PROGRESS:Update D] 15s - 38.00% iterations (1.554e-06)
[PROGRESS:Update D] 15s - 39.00% iterations (1.554e-06)
[PROGRESS:Update D] 16s - 40.00% iterations (1.554e-06)
[PROGRESS:Update D] 16s - 41.00% iterations (1.554e-06)
[PROGRESS:Update D] 17s - 42.00% iterations (1.554e-06)
[PROGRESS:Update D] 17s - 43.00% iterations (1.554e-06)
[PROGRESS:Update D] 17s - 44.00% iterations (1.554e-06)
[PROGRESS:Update D] 18s - 45.00% iterations (1.554e-06)
[PROGRESS:Update D] 18s - 46.00% iterations (1.554e-06)
[PROGRESS:Update D] 19s - 47.00% iterations (1.554e-06)
[PROGRESS:Update D] 19s - 48.00% iterations (1.554e-06)
[PROGRESS:Update D] 19s - 49.00% iterations (1.554e-06)
[PROGRESS:Update D] 20s - 50.00% iterations (1.554e-06)
[PROGRESS:Update D] 20s - 51.00% iterations (1.554e-06)
[PROGRESS:Update D] 20s - 52.00% iterations (1.554e-06)
[PROGRESS:Update D] 21s - 53.00% iterations (1.554e-06)
[PROGRESS:Update D] 21s - 54.00% iterations (1.554e-06)
[PROGRESS:Update D] 22s - 55.00% iterations (1.554e-06)
[PROGRESS:Update D] 22s - 56.00% iterations (1.554e-06)
[PROGRESS:Update D] 22s - 57.00% iterations (1.554e-06)
[PROGRESS:Update D] 23s - 58.00% iterations (1.554e-06)
[PROGRESS:Update D] 23s - 59.00% iterations (1.554e-06)
[PROGRESS:Update D] 24s - 60.00% iterations (1.554e-06)
[PROGRESS:Update D] 24s - 61.00% iterations (1.554e-06)
[PROGRESS:Update D] 24s - 62.00% iterations (1.554e-06)
[PROGRESS:Update D] 25s - 63.00% iterations (1.554e-06)
[PROGRESS:Update D] 25s - 64.00% iterations (1.554e-06)
[PROGRESS:Update D] 26s - 65.00% iterations (1.554e-06)
[PROGRESS:Update D] 26s - 66.00% iterations (1.554e-06)
[PROGRESS:Update D] 26s - 67.00% iterations (1.554e-06)
[PROGRESS:Update D] 27s - 68.00% iterations (1.554e-06)
[PROGRESS:Update D] 27s - 69.00% iterations (1.554e-06)
[PROGRESS:Update D] 28s - 70.00% iterations (1.554e-06)
[PROGRESS:Update D] 28s - 71.00% iterations (1.554e-06)
[PROGRESS:Update D] 28s - 72.00% iterations (1.554e-06)
[PROGRESS:Update D] 29s - 73.00% iterations (1.554e-06)
[PROGRESS:Update D] 29s - 74.00% iterations (1.554e-06)
[PROGRESS:Update D] 30s - 75.00% iterations (1.554e-06)
[PROGRESS:Update D] 30s - 76.00% iterations (1.554e-06)
[PROGRESS:Update D] 30s - 77.00% iterations (1.554e-06)
[PROGRESS:Update D] 31s - 78.00% iterations (1.554e-06)
[PROGRESS:Update D] 31s - 79.00% iterations (1.554e-06)
[PROGRESS:Update D] 32s - 80.00% iterations (1.554e-06)
[PROGRESS:Update D] 32s - 81.00% iterations (1.554e-06)
[PROGRESS:Update D] 32s - 82.00% iterations (1.554e-06)
[PROGRESS:Update D] 33s - 83.00% iterations (1.554e-06)
[PROGRESS:Update D] 33s - 84.00% iterations (1.554e-06)
[PROGRESS:Update D] 34s - 85.00% iterations (1.554e-06)
[PROGRESS:Update D] 34s - 86.00% iterations (1.554e-06)
[PROGRESS:Update D] 34s - 87.00% iterations (1.554e-06)
[PROGRESS:Update D] 35s - 88.00% iterations (1.554e-06)
[PROGRESS:Update D] 35s - 89.00% iterations (1.554e-06)
[PROGRESS:Update D] 36s - 90.00% iterations (1.554e-06)
[PROGRESS:Update D] 36s - 91.00% iterations (1.554e-06)
[PROGRESS:Update D] 36s - 92.00% iterations (1.554e-06)
[PROGRESS:Update D] 37s - 93.00% iterations (1.554e-06)
[PROGRESS:Update D] 37s - 94.00% iterations (1.554e-06)
[PROGRESS:Update D] 38s - 95.00% iterations (1.554e-06)
[PROGRESS:Update D] 38s - 96.00% iterations (1.554e-06)
[PROGRESS:Update D] 38s - 97.00% iterations (1.554e-06)
[PROGRESS:Update D] 39s - 98.00% iterations (1.554e-06)
[PROGRESS:Update D] 39s - 99.00% iterations (1.554e-06)
[INFO:Update D] update did not converge
[INFO:Update D]: 100 iterations
[DEBUG:DICODILE] Objective (d) : 3.141e+06 (45s)
[INFO:DICODILE] - CD iterations 10 / 100 (996s)
[DEBUG:DICODILE] lambda = 4.763e+00
[INFO:DICOD-10] converged in 29.556s (24.876s) with 206477 iterations (44748 updates).
[DEBUG:DICODILE] Objective (z) : 3.140e+06 (36s)
[PROGRESS:Update D] 1s - 2.00% iterations (3.284e-05)
[PROGRESS:Update D] 2s - 3.00% iterations (1.642e-05)
[PROGRESS:Update D] 2s - 4.00% iterations (1.642e-05)
[PROGRESS:Update D] 3s - 5.00% iterations (1.642e-05)
[PROGRESS:Update D] 3s - 6.00% iterations (1.642e-05)
[PROGRESS:Update D] 3s - 7.00% iterations (1.642e-05)
[PROGRESS:Update D] 4s - 8.00% iterations (1.642e-05)
[PROGRESS:Update D] 4s - 9.00% iterations (1.642e-05)
[PROGRESS:Update D] 5s - 10.00% iterations (1.642e-05)
[PROGRESS:Update D] 6s - 11.00% iterations (8.674e-07)
[PROGRESS:Update D] 6s - 12.00% iterations (8.674e-07)
[PROGRESS:Update D] 7s - 13.00% iterations (8.674e-07)
[PROGRESS:Update D] 7s - 14.00% iterations (4.337e-07)
[PROGRESS:Update D] 8s - 15.00% iterations (2.169e-07)
[PROGRESS:Update D] 8s - 16.00% iterations (2.169e-07)
[PROGRESS:Update D] 9s - 17.00% iterations (1.084e-07)
[PROGRESS:Update D] 9s - 18.00% iterations (5.422e-08)
[PROGRESS:Update D] 10s - 19.00% iterations (2.711e-08)
[PROGRESS:Update D] 10s - 20.00% iterations (1.355e-08)
[PROGRESS:Update D] 11s - 21.00% iterations (1.355e-08)
[PROGRESS:Update D] 11s - 22.00% iterations (6.777e-09)
[PROGRESS:Update D] 12s - 23.00% iterations (1.432e-09)
[PROGRESS:Update D] 13s - 24.00% iterations (1.432e-09)
[PROGRESS:Update D] 13s - 25.00% iterations (1.432e-09)
[INFO:Update D]: 26 iterations
[DEBUG:DICODILE] Objective (d) : 3.140e+06 (19s)
[INFO:DICODILE] - CD iterations 11 / 100 (1057s)
[DEBUG:DICODILE] lambda = 4.763e+00
[INFO:DICOD-10] converged in 24.468s (20.313s) with 207850 iterations (37604 updates).
[DEBUG:DICODILE] Objective (z) : 3.140e+06 (30s)
[PROGRESS:Update D] 1s - 3.00% iterations (7.161e-08)
[PROGRESS:Update D] 2s - 4.00% iterations (7.161e-08)
[PROGRESS:Update D] 2s - 5.00% iterations (7.161e-08)
[PROGRESS:Update D] 2s - 6.00% iterations (7.161e-08)
[PROGRESS:Update D] 3s - 7.00% iterations (7.161e-08)
[PROGRESS:Update D] 3s - 8.00% iterations (7.161e-08)
[PROGRESS:Update D] 4s - 9.00% iterations (7.161e-08)
[PROGRESS:Update D] 4s - 10.00% iterations (7.161e-08)
[PROGRESS:Update D] 4s - 11.00% iterations (7.161e-08)
[PROGRESS:Update D] 5s - 12.00% iterations (7.161e-08)
[PROGRESS:Update D] 5s - 13.00% iterations (7.161e-08)
[PROGRESS:Update D] 6s - 14.00% iterations (7.161e-08)
[PROGRESS:Update D] 6s - 15.00% iterations (7.161e-08)
[PROGRESS:Update D] 6s - 16.00% iterations (7.161e-08)
[PROGRESS:Update D] 7s - 17.00% iterations (7.161e-08)
[PROGRESS:Update D] 7s - 18.00% iterations (7.161e-08)
[PROGRESS:Update D] 8s - 19.00% iterations (7.161e-08)
[PROGRESS:Update D] 8s - 20.00% iterations (7.161e-08)
[PROGRESS:Update D] 8s - 21.00% iterations (7.161e-08)
[PROGRESS:Update D] 9s - 22.00% iterations (7.161e-08)
[PROGRESS:Update D] 9s - 23.00% iterations (7.161e-08)
[PROGRESS:Update D] 10s - 24.00% iterations (7.161e-08)
[PROGRESS:Update D] 10s - 25.00% iterations (7.161e-08)
[PROGRESS:Update D] 10s - 26.00% iterations (7.161e-08)
[PROGRESS:Update D] 11s - 27.00% iterations (7.161e-08)
[PROGRESS:Update D] 11s - 28.00% iterations (7.161e-08)
[PROGRESS:Update D] 12s - 29.00% iterations (7.161e-08)
[PROGRESS:Update D] 12s - 30.00% iterations (7.161e-08)
[PROGRESS:Update D] 12s - 31.00% iterations (7.161e-08)
[PROGRESS:Update D] 13s - 32.00% iterations (7.161e-08)
[PROGRESS:Update D] 13s - 33.00% iterations (7.161e-08)
[PROGRESS:Update D] 14s - 34.00% iterations (7.161e-08)
[PROGRESS:Update D] 14s - 35.00% iterations (7.161e-08)
[PROGRESS:Update D] 14s - 36.00% iterations (7.161e-08)
[PROGRESS:Update D] 15s - 37.00% iterations (7.161e-08)
[PROGRESS:Update D] 15s - 38.00% iterations (7.161e-08)
[PROGRESS:Update D] 15s - 39.00% iterations (7.161e-08)
[PROGRESS:Update D] 16s - 40.00% iterations (7.161e-08)
[PROGRESS:Update D] 16s - 41.00% iterations (7.161e-08)
[PROGRESS:Update D] 17s - 42.00% iterations (7.161e-08)
[PROGRESS:Update D] 17s - 43.00% iterations (7.161e-08)
[PROGRESS:Update D] 17s - 44.00% iterations (7.161e-08)
[PROGRESS:Update D] 18s - 45.00% iterations (7.161e-08)
[PROGRESS:Update D] 18s - 46.00% iterations (7.161e-08)
[PROGRESS:Update D] 19s - 47.00% iterations (7.161e-08)
[PROGRESS:Update D] 19s - 48.00% iterations (7.161e-08)
[PROGRESS:Update D] 19s - 49.00% iterations (7.161e-08)
[PROGRESS:Update D] 20s - 50.00% iterations (7.161e-08)
[PROGRESS:Update D] 20s - 51.00% iterations (7.161e-08)
[PROGRESS:Update D] 21s - 52.00% iterations (7.161e-08)
[PROGRESS:Update D] 21s - 53.00% iterations (7.161e-08)
[PROGRESS:Update D] 21s - 54.00% iterations (7.161e-08)
[PROGRESS:Update D] 22s - 55.00% iterations (7.161e-08)
[PROGRESS:Update D] 22s - 56.00% iterations (7.161e-08)
[PROGRESS:Update D] 23s - 57.00% iterations (7.161e-08)
[PROGRESS:Update D] 23s - 58.00% iterations (7.161e-08)
[PROGRESS:Update D] 23s - 59.00% iterations (7.161e-08)
[PROGRESS:Update D] 24s - 60.00% iterations (7.161e-08)
[PROGRESS:Update D] 24s - 61.00% iterations (7.161e-08)
[PROGRESS:Update D] 25s - 62.00% iterations (7.161e-08)
[PROGRESS:Update D] 25s - 63.00% iterations (7.161e-08)
[PROGRESS:Update D] 25s - 64.00% iterations (7.161e-08)
[PROGRESS:Update D] 26s - 65.00% iterations (7.161e-08)
[PROGRESS:Update D] 26s - 66.00% iterations (7.161e-08)
[PROGRESS:Update D] 27s - 67.00% iterations (7.161e-08)
[PROGRESS:Update D] 27s - 68.00% iterations (7.161e-08)
[PROGRESS:Update D] 27s - 69.00% iterations (7.161e-08)
[PROGRESS:Update D] 28s - 70.00% iterations (7.161e-08)
[PROGRESS:Update D] 28s - 71.00% iterations (7.161e-08)
[PROGRESS:Update D] 29s - 72.00% iterations (7.161e-08)
[PROGRESS:Update D] 29s - 73.00% iterations (7.161e-08)
[PROGRESS:Update D] 29s - 74.00% iterations (7.161e-08)
[PROGRESS:Update D] 30s - 75.00% iterations (7.161e-08)
[PROGRESS:Update D] 30s - 76.00% iterations (7.161e-08)
[PROGRESS:Update D] 31s - 77.00% iterations (7.161e-08)
[PROGRESS:Update D] 31s - 78.00% iterations (7.161e-08)
[PROGRESS:Update D] 31s - 79.00% iterations (7.161e-08)
[PROGRESS:Update D] 32s - 80.00% iterations (7.161e-08)
[PROGRESS:Update D] 32s - 81.00% iterations (7.161e-08)
[PROGRESS:Update D] 33s - 82.00% iterations (7.161e-08)
[PROGRESS:Update D] 33s - 83.00% iterations (7.161e-08)
[PROGRESS:Update D] 33s - 84.00% iterations (7.161e-08)
[PROGRESS:Update D] 34s - 85.00% iterations (7.161e-08)
[PROGRESS:Update D] 34s - 86.00% iterations (7.161e-08)
[PROGRESS:Update D] 35s - 87.00% iterations (7.161e-08)
[PROGRESS:Update D] 35s - 88.00% iterations (7.161e-08)
[PROGRESS:Update D] 35s - 89.00% iterations (7.161e-08)
[PROGRESS:Update D] 36s - 90.00% iterations (7.161e-08)
[PROGRESS:Update D] 36s - 91.00% iterations (7.161e-08)
[PROGRESS:Update D] 37s - 92.00% iterations (7.161e-08)
[PROGRESS:Update D] 37s - 93.00% iterations (7.161e-08)
[PROGRESS:Update D] 37s - 94.00% iterations (7.161e-08)
[PROGRESS:Update D] 38s - 95.00% iterations (7.161e-08)
[PROGRESS:Update D] 38s - 96.00% iterations (7.161e-08)
[PROGRESS:Update D] 39s - 97.00% iterations (7.161e-08)
[PROGRESS:Update D] 39s - 98.00% iterations (7.161e-08)
[PROGRESS:Update D] 39s - 99.00% iterations (7.161e-08)
[INFO:Update D] update did not converge
[INFO:Update D]: 100 iterations
[DEBUG:DICODILE] Objective (d) : 3.140e+06 (45s)
[INFO:DICODILE] - CD iterations 12 / 100 (1138s)
[DEBUG:DICODILE] lambda = 4.763e+00
[INFO:DICOD-10] converged in 8.048s (6.570s) with 54078 iterations (11741 updates).
[DEBUG:DICODILE] Objective (z) : 3.140e+06 (14s)
[PROGRESS:Update D] 1s - 3.00% iterations (7.161e-06)
[PROGRESS:Update D] 2s - 4.00% iterations (7.161e-06)
[PROGRESS:Update D] 2s - 5.00% iterations (7.161e-06)
[PROGRESS:Update D] 2s - 6.00% iterations (7.161e-06)
[PROGRESS:Update D] 3s - 7.00% iterations (7.161e-06)
[PROGRESS:Update D] 3s - 8.00% iterations (7.161e-06)
[PROGRESS:Update D] 4s - 9.00% iterations (7.161e-06)
[PROGRESS:Update D] 4s - 10.00% iterations (7.161e-06)
[PROGRESS:Update D] 4s - 11.00% iterations (7.161e-06)
[PROGRESS:Update D] 5s - 12.00% iterations (7.161e-06)
[PROGRESS:Update D] 5s - 13.00% iterations (7.161e-06)
[PROGRESS:Update D] 6s - 14.00% iterations (7.161e-06)
[PROGRESS:Update D] 6s - 15.00% iterations (7.161e-06)
[PROGRESS:Update D] 6s - 16.00% iterations (7.161e-06)
[PROGRESS:Update D] 7s - 17.00% iterations (7.161e-06)
[PROGRESS:Update D] 7s - 18.00% iterations (7.161e-06)
[PROGRESS:Update D] 8s - 19.00% iterations (7.161e-06)
[PROGRESS:Update D] 8s - 20.00% iterations (7.161e-06)
[PROGRESS:Update D] 8s - 21.00% iterations (7.161e-06)
[PROGRESS:Update D] 9s - 22.00% iterations (7.161e-06)
[PROGRESS:Update D] 9s - 23.00% iterations (7.161e-06)
[PROGRESS:Update D] 10s - 24.00% iterations (7.161e-06)
[PROGRESS:Update D] 10s - 25.00% iterations (7.161e-06)
[PROGRESS:Update D] 10s - 26.00% iterations (7.161e-06)
[PROGRESS:Update D] 11s - 27.00% iterations (7.161e-06)
[PROGRESS:Update D] 11s - 28.00% iterations (7.161e-06)
[PROGRESS:Update D] 12s - 29.00% iterations (7.161e-06)
[PROGRESS:Update D] 12s - 30.00% iterations (7.161e-06)
[PROGRESS:Update D] 13s - 31.00% iterations (7.161e-06)
[PROGRESS:Update D] 13s - 32.00% iterations (7.161e-06)
[PROGRESS:Update D] 13s - 33.00% iterations (7.161e-06)
[PROGRESS:Update D] 14s - 34.00% iterations (7.161e-06)
[PROGRESS:Update D] 14s - 35.00% iterations (7.161e-06)
[PROGRESS:Update D] 15s - 36.00% iterations (7.161e-06)
[PROGRESS:Update D] 15s - 37.00% iterations (7.161e-06)
[PROGRESS:Update D] 15s - 38.00% iterations (7.161e-06)
[PROGRESS:Update D] 16s - 39.00% iterations (7.161e-06)
[PROGRESS:Update D] 16s - 40.00% iterations (7.161e-06)
[PROGRESS:Update D] 17s - 41.00% iterations (7.161e-06)
[PROGRESS:Update D] 17s - 42.00% iterations (1.513e-06)
[PROGRESS:Update D] 18s - 43.00% iterations (7.567e-07)
[PROGRESS:Update D] 19s - 44.00% iterations (1.599e-07)
[PROGRESS:Update D] 19s - 45.00% iterations (1.599e-07)
[PROGRESS:Update D] 20s - 46.00% iterations (3.379e-08)
[PROGRESS:Update D] 20s - 47.00% iterations (1.690e-08)
[PROGRESS:Update D] 21s - 48.00% iterations (8.449e-09)
[PROGRESS:Update D] 22s - 49.00% iterations (4.224e-09)
[INFO:Update D]: 50 iterations
[DEBUG:DICODILE] Objective (d) : 3.140e+06 (28s)
[INFO:DICODILE] - CD iterations 13 / 100 (1187s)
[DEBUG:DICODILE] lambda = 4.763e+00
[INFO:DICOD-10] converged in 19.308s (16.402s) with 128506 iterations (30084 updates).
[DEBUG:DICODILE] Objective (z) : 3.139e+06 (25s)
[PROGRESS:Update D] 1s - 3.00% iterations (8.927e-08)
[PROGRESS:Update D] 2s - 4.00% iterations (8.927e-08)
[PROGRESS:Update D] 2s - 5.00% iterations (8.927e-08)
[PROGRESS:Update D] 2s - 6.00% iterations (8.927e-08)
[PROGRESS:Update D] 3s - 7.00% iterations (8.927e-08)
[PROGRESS:Update D] 3s - 8.00% iterations (8.927e-08)
[PROGRESS:Update D] 4s - 9.00% iterations (8.927e-08)
[PROGRESS:Update D] 4s - 10.00% iterations (8.927e-08)
[INFO:Update D]: 11 iterations
[DEBUG:DICODILE] Objective (d) : 3.139e+06 (12s)
[INFO:DICODILE] - CD iterations 14 / 100 (1230s)
[DEBUG:DICODILE] lambda = 4.763e+00
[INFO:DICOD-10] converged in 1.068s (0.760s) with 12321 iterations (1106 updates).
[DEBUG:DICODILE] Objective (z) : 3.139e+06 (7s)
[PROGRESS:Update D] 5s - 1.00% iterations (2.519e-06)
[INFO:Update D]: 2 iterations
[DEBUG:DICODILE] Objective (d) : 3.139e+06 (15s)
[INFO:DICODILE] Converged after 15 iteration, (dz, du) = 8.570e-08, 3.801e-08
[INFO:DICOD-10] converged in 2.532s (2.049s) with 19792 iterations (3400 updates).
[INFO:DICODILE] Finished in 1171s
[DICOD] final cost : [3328083.483032226, 3322241.735583827, 3288733.289882077, 3226087.2368888445, 3217977.3225730993, 3209002.832323461, 3205766.75786955, 3199859.673789586, 3195043.6056792084, 3187000.9523876817, 3178041.737437436, 3167250.024605673, 3156906.526775885, 3150609.1374183507, 3145849.8418757915, 3143956.091590614, 3142205.7589950897, 3141334.725412146, 3141261.1352075525, 3141256.570167604, 3140617.57811235, 3140244.1624557, 3139956.6709456914, 3139694.6416852437, 3139681.5124197137, 3139674.974150443, 3139567.4767157435, 3139418.4867491154, 3139418.4208492306, 3139418.151812008, 3139418.032470725, 3139417.033604104]
Let’s compare the initially generated random patches in D_init with the atoms in D_hat recovered with dicodile.
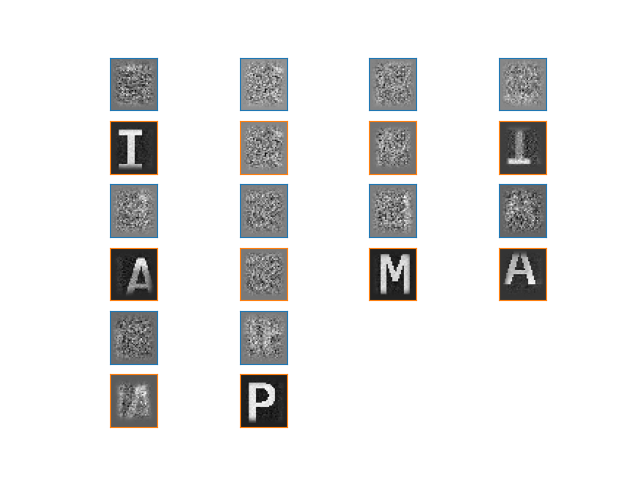
<Figure size 640x480 with 24 Axes>
Now we will reconstruct the image from z_hat and D_hat.
Let’s plot the reconstructed image X_hat together with the original image X_original and the noisy image X that was input to dicodile.
f, (ax1, ax2, ax3) = plt.subplots(3, 1, figsize=[6.4, 8])
ax1.imshow(X_original[190:490, 250:750], cmap='gray')
ax1.set_title('Original image')
ax1.axis('off')
ax2.imshow(X[0][190:490, 250:750], cmap='gray')
ax2.set_title('Noisy image')
ax2.axis('off')
ax3.imshow(X_hat[0][190:490, 250:750], cmap='gray')
ax3.set_title('Recovered image')
ax3.axis('off')
plt.tight_layout()
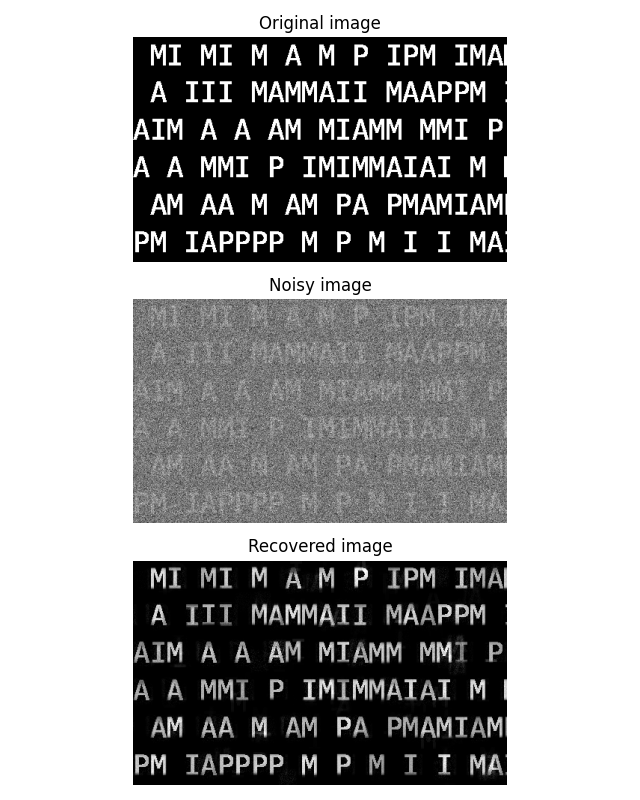
Total running time of the script: ( 21 minutes 27.263 seconds)